In today’s data-driven world, databases are at the core of almost every application—whether it’s a mobile app, a desktop tool, or a web-based service. Among the many database systems available, SQLite database stands out for its simplicity, portability, and minimal setup requirements. Whether you're a beginner learning SQL for the first time or a developer building a small-scale application, SQLite offers a perfect starting point.
Unlike traditional database systems that require a dedicated server and complex configuration, SQLite is a lightweight, file-based database engine that requires zero setup and minimal overhead. You can start creating and managing a database for SQLite with just a few lines of code or even directly from your terminal.
In this comprehensive guide, we’ll walk you through the essentials of getting started with SQLite—from setting it up and creating your first tables to understanding SQLite data types and mastering the most important SQLite commands. By the end of this article, you'll be equipped to build and manage simple, yet powerful databases efficiently.
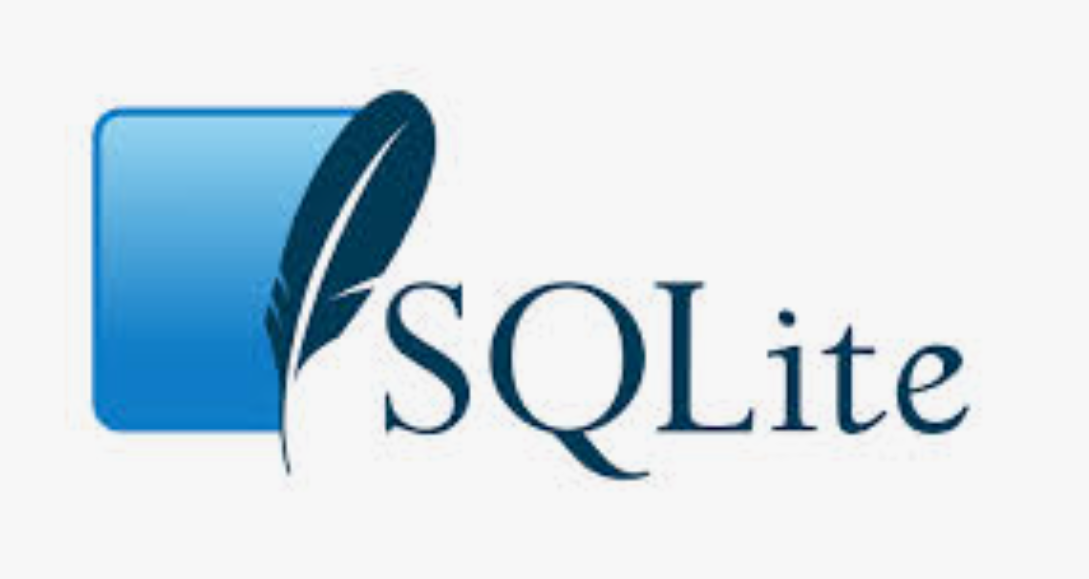
What is SQLite?
SQLite is an open-source, self-contained, serverless SQL database engine that stores data in a single cross-platform file. Unlike traditional relational databases such as MySQL or PostgreSQL, SQLite does not require a separate server process or system to operate. This makes it incredibly lightweight and easy to use, especially for developers who want to embed a database directly into their applications.
It is written in C and is widely regarded for its reliability, efficiency, and small footprint. In fact, SQLite db is so stable and portable that it's used by major operating systems, web browsers, and mobile applications—including iOS and Android.
Also Read: Common Deep Learning Interview Questions and How to Answer Them
Key Features of SQLite:
- Zero Configuration: No setup or installation required to start using a database.
- Cross-Platform: Works on Windows, macOS, Linux, Android, iOS, and more.
- Single Database File: Entire database is stored in one file, making it easy to manage, back up, and move.
- ACID Compliant: Supports atomic commit and rollback, ensuring data integrity.
- Embedded Database: Ideal for applications that need a simple, local database without the complexity of a server.
Whether you're building a prototype, learning SQL, or developing a lightweight app, SQLite is often the first choice for its ease of use and performance in small to medium-sized data scenarios.
Why Choose SQLite Database?
When selecting a database engine, it's important to consider the size and scope of your project. While enterprise applications often require powerful server-based databases like PostgreSQL or MySQL, many small to medium-scale projects benefit more from a simple, lightweight solution. That’s where the SQLite shines.
Advantages of Using SQLite:
- Simplicity and Ease of Use
- No need to install or configure a server.
- Just create a database SQLite and start running queries.
- Perfect for beginners learning SQL without complex setup steps.
- No need to install or configure a server.
- Lightweight and Fast
- Minimal disk and memory usage.
- Because everything is stored in a single file, it loads and performs faster in smaller applications.
- Minimal disk and memory usage.
- Portable and Cross-Platform
- Your entire database is a single .sqlite or .db file that can be easily moved or shared.
- Works on nearly every operating system without any changes.
- Your entire database is a single .sqlite or .db file that can be easily moved or shared.
- Serverless Architecture
- No client-server model; the application reads and writes directly to the database file.
- Ideal for embedded systems, local applications, and mobile devices.
- No client-server model; the application reads and writes directly to the database file.
- Reliable and Well-Tested
- Used by giants like Apple, Google, Adobe, and Mozilla in various products.
- Fully compliant with the SQL-92 standard and widely trusted in production environments.
- Used by giants like Apple, Google, Adobe, and Mozilla in various products.
- Open Source and Free
- SQLite is free to use for commercial and personal projects.
- Its open-source nature allows for transparency and community support
- SQLite is free to use for commercial and personal projects.
When to Use SQLite:
- Prototyping and proof-of-concept apps.
- Learning SQL basics with hands-on practice.
- Local storage in mobile or desktop apps.
- Applications that require simple, embedded databases without multi-user access.
SQLite may not be the right choice for large-scale applications needing concurrent access or high-volume write operations, but for many use cases, it offers the perfect balance of simplicity and functionality.
Setting Up SQLite
Getting started with SQLite is refreshingly simple. Unlike traditional database systems that need a server setup, SQLite is a zero-configuration database. You can start using it almost immediately—no installation wizards or admin panels required.
Installing SQLite
Depending on your operating system, here’s how you can set it up:
Windows
- Go to the official SQLite download page.
- Download the precompiled binaries for Windows (sqlite-tools).
- Extract the zip file and place it in a folder (e.g., C:\sqlite).
- Add the folder path to your system’s environment variables to run sqlite3 from the terminal.
macOS
SQLite is pre-installed on most macOS systems. To verify:
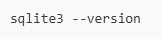
If not installed, use Homebrew:
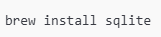
Linux (Ubuntu/Debian)
Use APT to install:

Creating a Database for SQLite
Once installed, creating a new SQLite db is easy:

This command:
- Opens the SQLite command-line shell.
- Creates a file named mydatabase.db if it doesn’t exist.
- Launches an interactive session where you can start using commands.
To exit the shell, type:

GUI Tools for SQLite (Optional)
If you prefer graphical interfaces over the terminal, here are some tools:
- DB Browser for SQLite (Free and open-source)
- SQLiteStudio
- VS Code SQLite Extensions
These tools allow you to browse tables, run queries, and visualize data without writing all commands manually.
With SQLite installed and your first database created, you're now ready to explore tables, data types, and commands.
Also Read: What is Gradient Descent in Deep Learning? A Beginner-Friendly Guide
SQLite Data Types Explained
One of the unique aspects of the SQLite db is its dynamic typing system. Unlike other relational databases that enforce strict data types for each column, SQLite uses a more flexible concept known as type affinity. This means that while you can declare a column to be of a certain data type, SQLite doesn't strictly enforce that type—it uses rules to determine how the data is stored and processed.
Core SQLite Data Types
SQLite supports the following five primary data types, also known as storage classes:
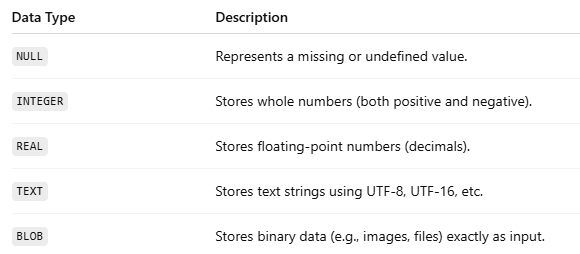
Example:
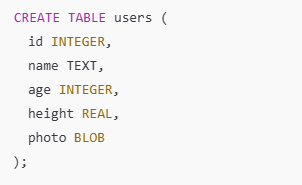
Type Affinity in SQLite
While you can define columns with various types (like VARCHAR(100) or BOOLEAN), SQLite maps them to one of the five storage classes using type affinity rules.
Here’s how some common SQL types are interpreted:
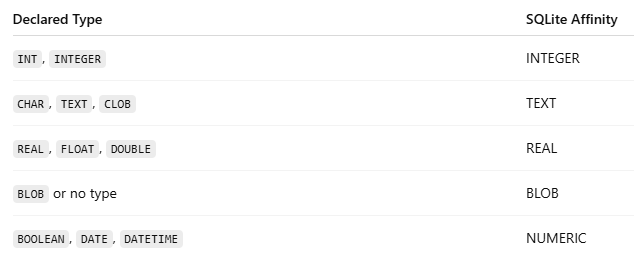
This flexible approach simplifies schema design but requires developers to be mindful of data validation at the application level.
Choosing the Right SQLite Data Types
Although SQLite lets you insert almost any value into any column, it's a good practice to choose the correct affinity:
- Use INTEGER for IDs, counters, and whole numbers.
- Use REAL for prices, measurements, or scientific data.
- Use TEXT for names, descriptions, and messages.
- Use BLOB only when dealing with binary content (like images or audio).
- Avoid relying on BOOLEAN, DATE, or DATETIME unless you handle conversion manually.
Understanding SQLite data types is essential for designing efficient and readable schemas. Now that you know how data is stored, let's dive into the most commonly used commands in SQLite.
Essential SQLite Commands
Now that you’ve set up your SQLite db and understood how data types in SQLite work, it’s time to interact with your data using commands. These SQL-based commands allow you to create, modify, and query your database.
Below are the most commonly used commands for beginners and intermediate users:
Creating Tables
Use the CREATE TABLE command to define a new table in your SQLite.

Inserting Data
Use the INSERT INTO command to add new rows:

Querying Data
Retrieve data using the SELECT command:
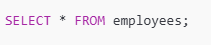
Filter results using WHERE:

Updating Records
Modify existing records using UPDATE:
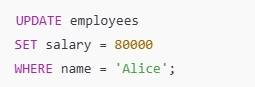
Deleting Records
Remove rows using DELETE:
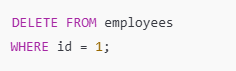
Dropping Tables
To permanently delete a table:
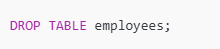
Viewing Tables and Schema
List all tables in your SQLite:
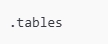
Display table schema (structure):

These basic commands are all you need to start building, modifying, and querying your database. As you grow more comfortable, you can explore advanced features like joins, indexing, and constraints.
Also Read: A Beginner’s Guide to Recurrent Neural Networks (RNN) in Deep Learning
Practical Example – Creating a Simple Student Database
To solidify your understanding of SQLite-commands, let’s walk through a practical example where we create and manage a simple SQLite for handling student information.
Step 1: Create the Database
First, open your terminal and start the SQLite shell by typing:
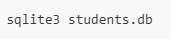
This creates a new database called students.db and opens the SQLite prompt.
Step 2: Create a Table

Here, we define a students table with various data types including INTEGER, TEXT, and REAL.
Step 3: Insert Student Records
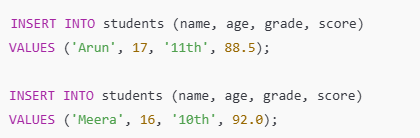
Step 4: Query the Table
To see all students:

To filter students by grade:
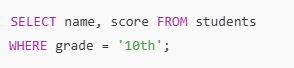
Step 5: Update a Record
Suppose Arun's score has been updated:
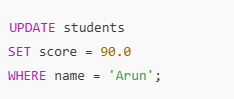
Step 6: Delete a Record
To delete a student record:
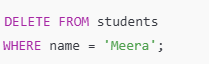
Step 7: Exit SQLite
Once you're done, type:

This real-world example demonstrates how simple and effective SQLite can be for managing structured data. Whether you're building a prototype or managing small datasets, SQLite offers all the essential features with minimal overhead.
Tips and Best Practices for Using SQLite
While the SQLite is lightweight and easy to use, following a few best practices can help you avoid common pitfalls and ensure optimal performance, especially as your application grows.
1. Choose the Right Data Types
Even though SQLite is flexible with data types, always assign a sensible type for each column using correct type affinity. This improves readability, ensures consistency, and helps with future migrations to other databases if needed.
2. Use Indexes Wisely
Indexes can speed up data retrieval:

But overusing them can slow down INSERT, UPDATE, and DELETE operations. Only index columns that are frequently queried.
3. Normalize Your Data
Keep your schema clean and normalized to reduce data redundancy. Use separate tables and relationships instead of storing everything in one table.
4. Use Parameterized Queries in Applications
If you're connecting SQLite with a programming language (like Python), avoid building SQL queries by string concatenation. Instead, use placeholders to prevent SQL injection:

5. Backup Your Database
Since SQLite stores everything in a single .db file, backup is as simple as copying the file. Always keep backup copies, especially before major updates.
6. Understand SQLite’s Limitations
SQLite is not designed for:
- High-concurrency, high-write applications
- Large-scale enterprise databases
Use it for mobile apps, prototypes, small to medium apps, or embedded systems.
7. Use .dump for Exporting and Importing
To export a database:

To import:

By following these practices, you'll make the most of SQLite’s simplicity while ensuring data integrity, maintainability, and performance.
Also Read: Everything You Need To Know About Optimizers in Deep Learning
Conclusion
Whether you're building a prototype, a mobile app, or learning SQL for the first time, the SQLite database is one of the best tools to start with. Its zero-configuration setup, portability, and fast performance make it a favorite among developers, educators, and data enthusiasts alike.
In this guide, we’ve covered:
- What SQLite is and why it's popular
- How to install and set up a database for SQLite
- Common SQLite commands for creating, reading, updating, and deleting data
- The flexible and powerful data types
- A practical student database example to solidify your understanding
- Best practices to make your work efficient and safe
As you become more comfortable with SQLite, you’ll discover just how versatile and powerful it can be, even for real-world applications. It’s not just a beginner’s tool—it’s a reliable engine for many production systems.
Ready to explore more? Try integrating SQLite with Python, Android, or web apps to take your skills to the next level.
Ready to transform your AI career? Join our expert-led courses at SkillCamper today and start your journey to success. Sign up now to gain in-demand skills from industry professionals. If you're a beginner, take the first step toward mastering Python! Check out this Full Stack Computer Vision Career Path- Beginner to get started with the basics and advance to complex topics at your own pace.
To stay updated with latest trends and technologies, to prepare specifically for interviews, make sure to read our detailed blogs:
How to Become a Data Analyst: A Step-by-Step Guide
How Business Intelligence Can Transform Your Business Operations
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Suspendisse varius enim in eros elementum tristique. Duis cursus, mi quis viverra.